How to create an RPA flow for Invoke Python script
Overview
In this tutorial, we will learn how to use the Invoke Python Script activity. We demonstrate a simple use case of invoking a python script that converts a given PDF into an image (PNG) file.
Components
- RPA
- RPA Management
- RPA Studio
- Python
- Poppler tool
- Installation instructions for Windows
Requirements
- You need to install the latest version of RPA Studio on your machine:
- Download the connection files
- Download RPA Installer from RPA Management. For this tutorial you need to install:
- IDE Studio
- Establish a connection between the RPA Studio and the RPA Management
- You can check the Infor RPA Studio User Guide for the details
Tutorial
Difficulty: Easy
Estimated completion time: 10 Minutes + 5 Minutes for the Preparations
1. Prepare Poppler and Python
Before we start building the RPA Flow, we need to ensure a few of the pre-requisites are satisfied.
Installing Poppler for Windows
For this tutorial, we are using a tool called Poppler to convert the PDFs to images. Poppler is a PDF rendering toolkit. Python libraries such as pdf2image and pdftotext rely on these Poppler tools to process PDF files.
Windows does not come with Poppler pre-installed, so you have to install it:
- Download the latest Windows Poppler binaries (e.g., from the unofficial Poppler for Windows releases by oschwartz) and extract the archive.
- Place the Poppler folder in a convenient location (for example,
C:\Poppler\
). - Then add the Poppler
bin
directory (e.g.C:\Poppler\bin
) to your system PATH environment variable. This allows Windows to find Poppler tools from any command prompt. - After updating PATH, open a new Command Prompt and run
pdftoppm -h
to confirm it’s working.
Python should be locally installed on the machine where the RPA Flow is supposed to run. Copy the following code in a text file and save it as PythonPDF.py. Note: The python script needs to be pointed towards the poppler/bin folder to function correctly.
Note: It’s best practice to have the script out of the working Project folder to avoid problems while invoking.
Create the folder to store the PDF to convert e.g. {“C:\RPA\PythonScript\PNG”}
import os
import sys
from pdf2image import convert_from_path
def convert_pdfs_to_png(input_dir):
# Get a list of PDF files in the input directory
pdf_files = [f for f in os.listdir(input_dir) if f.endswith('.pdf')]
output_dir = os.path.join(input_dir, "PNGs")
os.makedirs(output_dir, exist_ok=True)
# Convert each PDF file to PNG images
for pdf_file in pdf_files:
pdf_path = os.path.join(input_dir, pdf_file)
images = convert_from_path(pdf_path, poppler_path=r"C:\RPA\Scripts\poppler-0.68.0_x86\poppler-0.68.0\bin")
# Save each image as a PNG file
for i, image in enumerate(images):
image_path = os.path.join(output_dir, f"{pdf_file}_{i + 1}.png")
image.save(image_path, "PNG")
print(f"Converted {pdf_file} to PNG images.")
print("Conversion completed successfully.")
convert_pdfs_to_png(sys.argv[1])
2. Open RPA Studio
Open RPA Studio on your machine, and sign in, if required.
3. Create a new project
On the Home page click Create New Project and specify the following properties in the pop up window:
- Name: InvokePythonScriptConvert
- ProjectLocation: <default>
- Description: Invoke Python Script to convert a PDF to image.
- Language: VB <selected by default.
4. Define the Invoke Python Script activity
Drag and Drop the Invoke Python Script activity part of the programming category. You can find the list of activities by clicking Activities in the left-hand side bar or from the View menu in the top bar. Use search to find the activity you want.
The activity will allow you to Invoke a python script form a specific directory. We will configure it in the following way:
- Script Location: This property takes in the local path of the Python Script, so let’s create an variable called scriptPath of type string and default value e.g. “C:\RPA\PythonScript\PythonPDF.py“.
- Additional Arguments: This property takes in the path of PDFs that we want to convert. This could be path to a single PDF or a folder that contains the PDFs. Basically, this property sends the parameters to the method(s) defined in the Python Script. Let’s create the argument for it:
- called fileDirectory of type IEnumerable (System.Collections.Generic.IEnumerable<system.string>) (Variable type/Browse for Types) and default value pointing to the folder where the .pdf files are stored e.g. {“C:\RPA\PythonScript\PNG”}
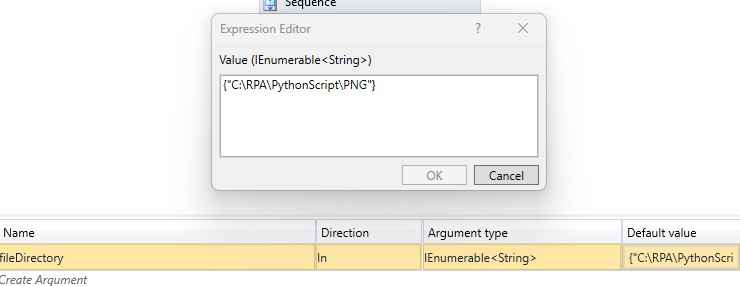
- Python Installation: If Python is installed in a non-standard location, the activity requires a path to the location. For this use case, we have Python in the default location.
- Requirements: This property takes in the path to a text file that contains the packages that are needed for the Python script. In this case, the text file only contains one package “pdf2image”. Let’s create the variable for the file called requirementPath of type string with default value pointing to e.g. “C:\RPA\PythonScript\requirements.txt”
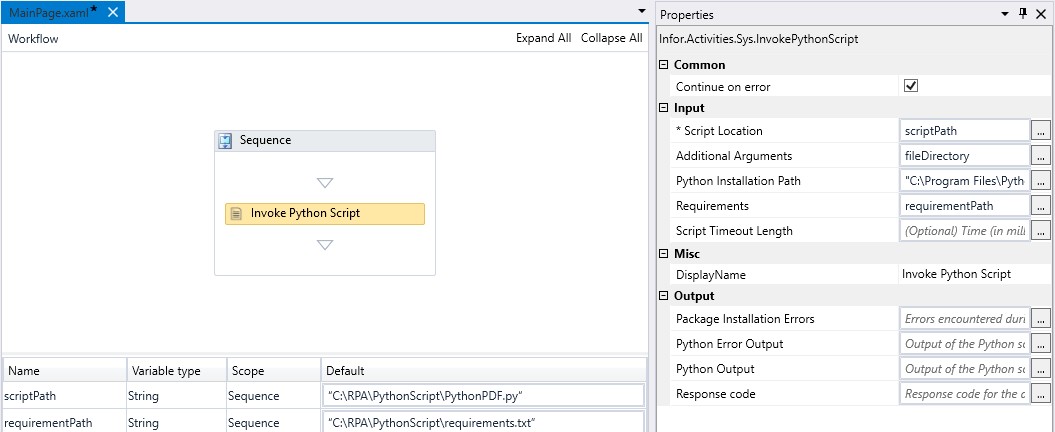
5. Edit the Python script
Let’s take a look at our Python Script and the change that is required to be made there.
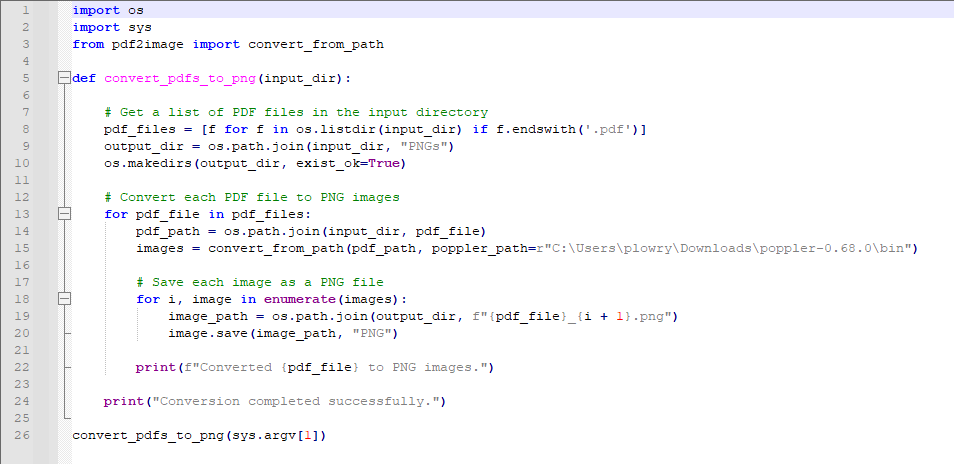
In the Python script, we would have to update the path to poppler\bin folder in the “poppler_path”.
6. Run the RPA Flow
Before you run the script, move a pdf to the C:\RPA\PythonScript\PNG folder for testing. Click Run to execute the RPA Flow, and the Activity will invoke the script, which will convert the PDF into images, and the images will be in the same folder as the PDFs, under “PNGs” sub-folder. Inside of the project folder a new folder called “script” will be created which will store the python script as well as the requirements.
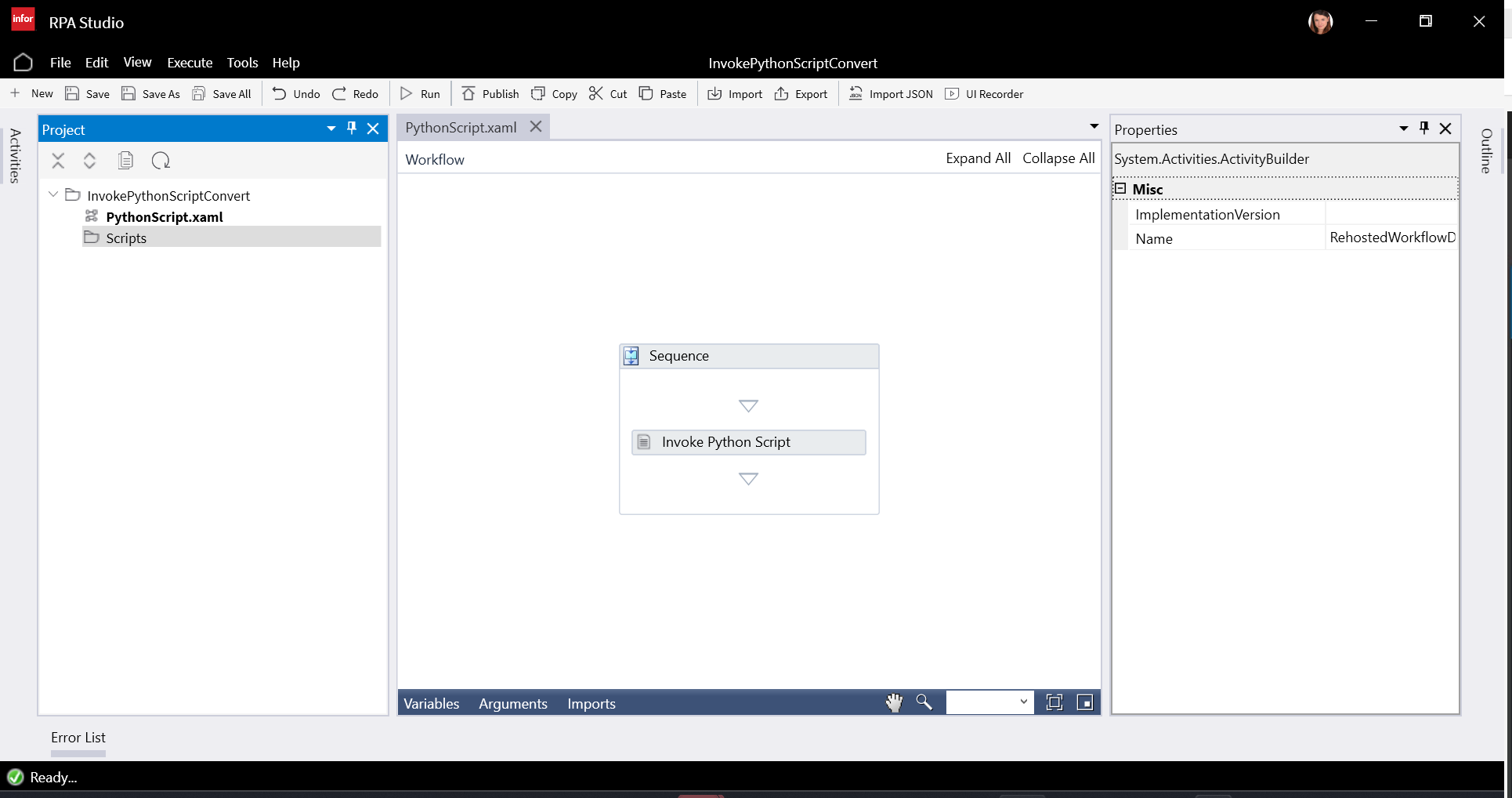